BALAD is an assembly-level programming language for an emulated virtual computer with a 15-bit word length. It is combined with a comprehensive debugging system that allows online program assembly, execution of programs and the insertion of breakpoints to allow suspension of programs during execution.
Instructions are machine-oriented, using integer and logical operations only. As a concession to the beginner, extra input/output instructions are provided for automatic Decimal to Binary conversion and the printing of Text Strings. These facilities will enable students to obtain a reasonable printout of their results quickly while concentrating their efforts on developing algorithms.
The following tells the story of how BALAD was first developed in the ’70s for teaching computer technicians the basic workings of a computer and my recent efforts to re-write BALAD to run on a PC. To complete the story I reach back to my first contact with the English Electric Deuce vacuum tube computer UTECOM in which I was privileged to write my first computer program in 1957. I ported the higher-level language GEORGE, used on UTECOM to BALAD to show how little computer resources are needed for a very useful program.
Today computers have become a throw-away item and there are very few technicians who would even know how to fix one. Most programmers think in terms of higher-level computer languages and have never taken the trouble to learn the very basics of how a computer works and what it does. The very convoluted and extensive CISC instruction sets of modern CPU’s do not make the study of assembler languages inviting. Nevertheless, I feel that beginning students in computing would benefit if they started by learning the machine instructions and structure of a simple von Neumann machine and had such a machine available to try out simple algorithms and seeing results immediately. BALAD is such a machine, providing for program entry, assembling and running code and in addition, provides a powerful debugger with breakpoint and tracing facilities. Learning programming and debugging in such a simple environment will be very useful for later work with higher-level language development tools.
In 1970, after completing the course work for my Master’s Degree in Computer Science, I was invited to design and give a course in computing as a post-certificate course for graduated technicians at the North Sydney Technical College (later TAFE) by one of my fellow Master’s student who was Head Teacher of the Electrical Engineering department at the college.
The college had recently acquired a Data General Nova minicomputer, which was top technology at the time, with 4 Accumulators and 16-bit word size. I was using a similar computer for my regular work at the CSR Research Laboratory. (The 2nd picture shows a Nova CPU with many MSI chips, which had just been developed by Fairchild Semiconductor).
The teachers at the college had been teaching computer programming, by simulating a simple single accumulator von Neumann computer with students sitting around a table. Each student took on the job of one part of the machine – Input, Memory, Instruction Decoder, Arithmetic Unit with the help of a mechanical calculator and Output. That way the execution of a program in the Memory could be followed by the group and the workings of the computer understood. The teachers at the college found the 4-accumulator Nova with its RISC instruction set too complex to teach the fundamentals of computing as they were used to, and I was asked if I could not produce something simpler. I was into computer emulation at the time, having read Turing’s paper, which stated that any Turing complete computing machine could emulate any other such computing machine. Having worked for my university work on a PDP-8, I had recently written the fastest PDP-8 emulator on a Nova as a competition project in the Data General Users Group.
For the college, I designed and implemented a very simple von Neumann type virtual computer to run on the Nova.
The BALAD computer is a one accumulator machine with an arithmetic unit for performing logical operations and 15 bit two’s complement arithmetic. The Memory has 512 15 bit words with addresses 0 to 777 (octal numbering). Memory words may contain instructions, addresses of other memory words or data.
At the start of every instruction cycle, the address in the Program Counter register (PC) is used by the computer to fetch an instruction from memory into the Instruction Decoder. The program counter is normally incremented by 1 at the end of an instruction cycle so that the next instruction is fetched from the next location in Memory. Only a jump instruction can break this sequence by loading the Program counter (PC) with a new address which points to some arbitrary point in the memory from where the next instruction will then be fetched.
The Instruction Decoder isolates bits 1 to 5 of the instruction and uses this as a number to distinguish between one of the 32 possible instructions. The instruction that has been identified is then executed. In a hardwired computer, this process involves setting various switches to allow data to flow from various registers to other registers.
For illustration two such switches are shown in the schematic above, which guide the result and carry values of an operation from the arithmetic unit either to the Accumulator and Carry for double operand instructions, ignore them for compare instructions or back to Memory and Carry for single operand instructions. The last 10 bits of the instruction are used to determine a memory reference address. This can again be thought of as setting a big switch that connects one data path to one of 512 words in memory.
The BALAD Manual contains a full description of the BALAD instruction set and how it is used to create a wide variety of assembler programs.
The original version of the BALAD emulator, Assembler and Debugger was written in Nova Assembler Language and was used by the teachers of the college and by me for the post-certificate course in computing. The workings of the computer underlying the BALAD emulator could be taught in a 90-minute evening lecture, leaving the rest of the evening for students to develop algorithms and enter programs via a teletype. The motivation of seeing results instantly was a huge factor in the success of that course. Two anecdotes are vivid memories from that time.
- A group of my student who was interested in music developed a data representation of music and wrote a BALAD program to interpret the frequency and duration of notes by having loops within loops – one for setting the duration of each cycle and the other for setting the length of the note. The activity in the Nova core memory when running the BALAD music program was such, that it could be picked up by an AM radio, which played the music in the BALAD data block. This was all worked out by the students without my help.
- Two students in another year worked for Westinghouse and were given the task of automating a machine for testing brake linings with a Nova computer by their employer. Although I did cover the Nova instruction set in my course, these two students felt more comfortable with BALAD and programed the brake lining test machine controller in that language in a minimum of time.
In 2021, I re-wrote BALAD as a Perl script in 4 weeks to run on a PC or Raspberry Pi, using the original lecture notes as a specification. I was inspired to do this by my grandson Felix, who was studying computing at school.
The picture shows an ARM CPU chip, which is used on a Raspberry Pi.
Since the BALAD instruction set only has ADD and SUBtract instructions for integer arithmetic and no MULtiply or DIVide instruction I showed Felix how to write a multiply routine, by adding the multiplier repeatedly to the accumulator while counting down the multiplicand until it becomes zero. This is not very efficient for large multiplicands, but it works well enough on modern fast computers. In BALAD it looks like this:
multiplier: 20 multiplcnd: 5 counter: 0 main: LDA multiplcnd STA counter CLR ACC loop: ADD multiplier DEC counter JNR loop PDN ACC ; print result on the terminal HLT
As an exercise, I also wanted to show Felix and my students how computers are used for displaying graphic images. For this purpose, I wrote a BALAD program to generate a picture of a Smiley using text-based graphics.
//=============================================\\ ||.................###########.................|| ||...........#######################...........|| ||........#########...........#########........|| ||......#######...................#######......|| ||....######.........................######....|| ||...####...............................####...|| ||..###...................................###..|| ||.###......##.....................##......###.|| ||###......####...................####......###|| ||###.....######.................######.....###|| ||##......######.................######......##|| ||##.......####...................####.......##|| ||##.........................................##|| ||##......##.........................##......##|| ||###....####.......................####....###|| ||###...##..####.................####..##...###|| ||.###.......########.......########.......###.|| ||..###..........###############..........###..|| ||...####..............###..............####...|| ||....######.........................######....|| ||......#######...................#######......|| ||........#########...........#########........|| ||...........#######################...........|| ||.................###########.................|| \\=============================== for FELIX ===//
A full description of the BALAD virtual computer, its instruction set and some of its inner workings can be found in the BALAD Manual in the Books section of this Blog. This also contains a full description of the BALAD assembler language and how to use it as well as a detailed description of the Debugger and its different instructions.
The complete BALAD system can be cloned from https://github.com/JohnWulff/balad. It can be used directly on all Linux and Mac OS X operating system computers. BALAD is a Perl program and to run BALAD on a Windows computer, you need to install either Strawberry Perl or Active State Perl from https://www.perl.org/get.html. BALAD works with both, although I prefer Active State Perl because it provides a more UNIX like environment. BALAD runs as a command-line program in a Command window. Both systems initially produce the following error message on Windows: Unable to get terminal size. To fix that, execute the following once:
set TERM=dumb
Early computers were largely seen as machines to do numerical calculations and were called ‘Number Crunchers’. UTECOM, which we will look at next was certainly a machine like that. To be up to the times in the ’70s I designed the BALAD instruction set to also allow a certain amount of text processing, which was recognised in the ’60s as being a major portion of the computing load of computers. Jobs like compiling higher-level languages into assembler code and assembling that into executable machine code are all text manipulations. BALAD is deemed to be a Turing complete instruction set and can do all these jobs. To prove this point, I wrote a compiler and interpreter in BALAD for the computer language GEORGE, which was used on UTECOM in the late ’50s.
UTECOM was a general-purpose vacuum-tube digital computer, with a serial organization and a 1Mhz clock rate. The word size was 32 bits, and the machine’s arithmetic units were capable of performing single, double, and mixed-precision binary integer arithmetic. Negative values were held in two’s complement form. A hardware unsigned integer multiplier and a signed integer divider were included.
Its primary memory consisted of 12 mercury-filled delay lines. These were “folded” with a quartz crystal transmitter and receiver at the top and a pair of acoustic mirrors at the bottom. The delay lines were situated in a temperature-controlled container called the mushroom.
Each delay line circulated 32 words of 32 bits each, making a total of 384 words (1536 bytes). Of the 384 words, 256 words (1024 bytes) were used for program memory and 128 words for data memory. This means that the program memory was of similar size as the BALAD memory of 512 x 15 bit words (1024 bytes). UTECOM just had an extra 50% of data memory, which made the storage of variables and other extras possible.
GEORGE is a nearly complete implementation of a Reverse Polish Notation (RPN) Calculator for double-precision integer arithmetic (30-bit). I did this with two aims in mind:
- To demonstrate what can be done with a minimal Turing complete instruction set computer and limited memory of 512 words (1024 bytes). Most of the demonstration programs I have supplied are toy programs to demonstrate various aspects of the instruction set. GEORGE (george.bl) is a full application with many of the capabilities of the calculator dc developed for early UNIX systems and still available in Linux systems. Other well-known RPN calculators are the HP-42S Scientific calculator introduced in 1988 and PCalc available on iPads and other mobile devices, which has an RPN mode. The main limitation of george.bl compared with these systems is the lack of floating-point arithmetic and the ability to use named variables. Only the lack of memory stops one from adding these capabilities.
- To show 21st-century students how computer languages developed in the early days of computing and the importance of the stack concept in computer languages.
Charles Leonard Hamblin was the professor of philosophy at the NSW University of Technology, which later became the University of NSW in 1955. Among his most well-known achievements in the area of computer science was the introduction (some sources also say invention) of the Reverse Polish Notation and the invention of the stack in computing.
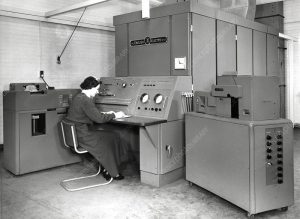
In the second half of the 1950s, he became active with UTECOM, the third computer available in Australia, which was a DEUCE computer produced by the English Electric company.
Charles Hamblin designed and implemented GEORGE, one of the first programming languages for UTECOM. GEORGE, which was based on Reverse Polish Notation, included the associated compiler (language translator), which translated the programs formulated in RPN notation into the machine language of the computer. In 1957 the GEORGE compiler was operational and I had the pleasure of attending Charles Hamblin’s philosophy lectures during the early part of my Electrical Engineering studies at UNSW. He explained the workings of GEORGE to us and the use of Reverse Polish Notation. I wrote my first computer program in this language and had it run on this very large machine.
Here are some extracts from the original GEORGE Programming and Operation Manual.
GEORGE, or the ‘General Order Generator’, is a program for DEUCE permitting mathematical problems to be presented to the machine in a simple “addressless” instruction language, here called “G-Code”. To use this code the programmer must learn a special method of writing mathematical formulae, known as “reverse Polish” notation. Once this is mastered, however, programming is considerably easier and quicker than by other methods. “G-Code” is a highly simplified and condensed instruction language. The program in G-Code in fact resembles a mathematical formula for the result required more than it does an orthodox machine program. In particular, the programmer never has to specify any “addresses” for numbers or instructions inside the machine.
As written, a program in G-Code is a sequence of mathematical symbols such as numerals, variables and arithmetical and other special signs; the symbols are transcribed to cards in a numerical code (on today’s computers a program is stored in a file in ASCII code – which is also a numerical code). Broadly, each symbol may be regarded as an individual “instruction” of the program.
To run GEORGE on a PC execute george.bl, or perl george.bl under Windows. Execute george.bl -h to print out the help text for GEORGE.